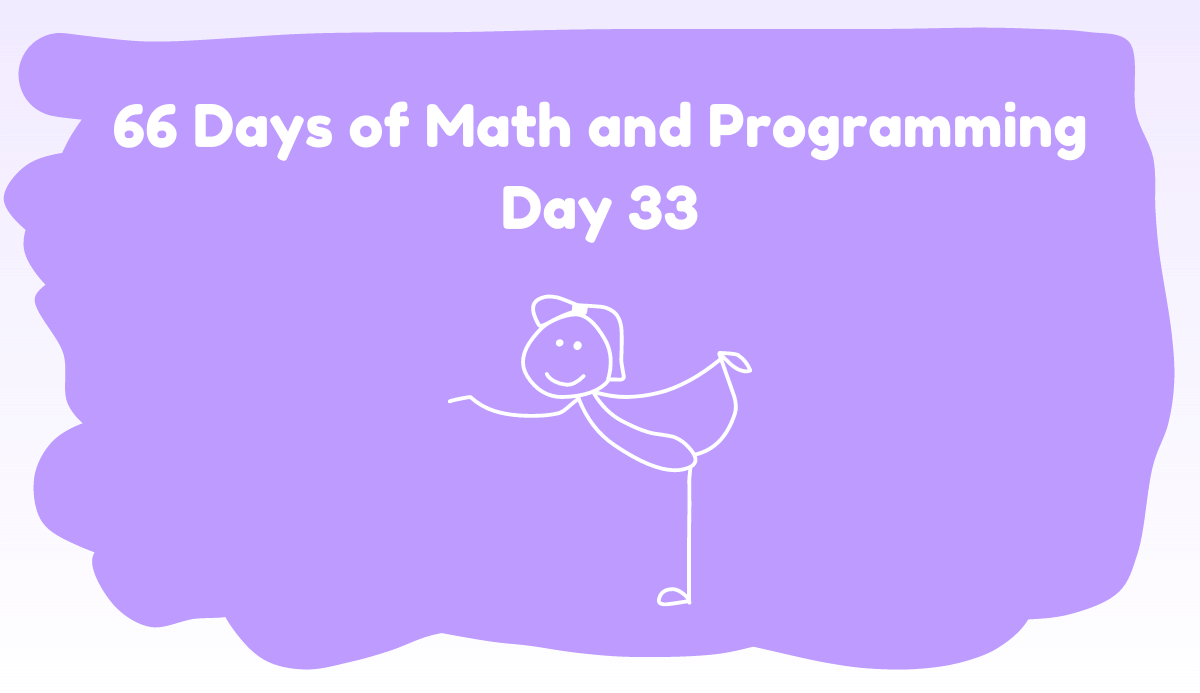
August 4, 2023
I’m keeping today’s blog post short yet insightful.
It’s gonna be all about the three Python habits I wish I learned in college.
Here they are:
Let’s dive in…
When I studied Python in college, anytime I had to write a string with a variable in the middle of it, I’d concatenate them together.
It looked something like this…
But that’s inefficient and takes up a lot of space in code. If you ever catch me doing it, punish me for it. Please.
Now I use f-strings instead because they feel more natural to write and look better in your scripts.
Here’s an example…
The first time I saw this, it made no sense. Why not just call your function at the end of your code, right?
Then I ran into an issue a few weeks ago…
While working on a script for my Dorky Email Automation project, I had to import a function from another script to pull a random item from my database.
But whenever I ran my code, this function seemed to always run without me calling it.
That’s when Detective Mike came out to play.
I discovered that when you import a function from a different Python script, the function automatically runs once you run the entire script.
That was a problem.
Until I learned about the ( if __name__ == “__main__” ) Python method.
It helps you only run the functions you want when you’re importing some from other scripts or modules.
I remember learning how to access text, CSV, and JSON files at the end of my Intro to Computer Science class.
It seemed super confusing because the professor kept talking about using .open() and .close() to access the files.
And he stressed that bad things could happen if you forget that close statement.
But look at me now…
I never have to use those close statements since I read and write files using “with” statements.
They automatically close the file after you exit the loop, which saves time, energy, and sanity.
I never learned about documenting code in the computer science class I took. It bit me in the butt a few weeks ago as a result.
I wrote a Python script for my Spotify Algorithm but didn’t use it for a few weeks.
When I returned to it, I completely forgot:
This is a major problem in the long run.
If you don’t understand what your code does, you’ll struggle to work with it in the future. That’s why I always document my code.
I’m never gonna forget why I wrote something again.